How to Create a class in PHP
Limited Time Offer!
For Less Than the Cost of a Starbucks Coffee, Access All DevOpsSchool Videos on YouTube Unlimitedly.
Master DevOps, SRE, DevSecOps Skills!
Interface:
An interface is like a class with nothing but abstract methods. All methods of an interface must be public. It is also possible to declare a constructor in an interface. It’s possible for interface to have constants(can not be overridden by a class/interface that inherits them). interface keyword is used to create an interface in php.
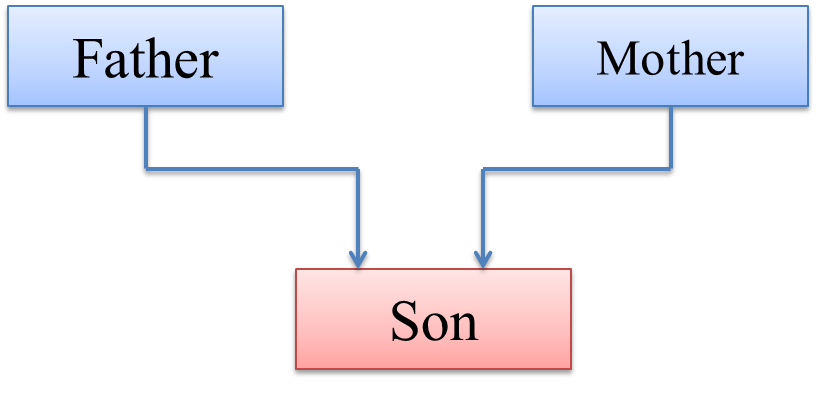
- Interface don’t have instance variables.
- All methods of an interface is abstract.
- All methods of an interface are automatically (by default) public.
- We can not use the private and protected specifiers when declaring member of an interface.
- We can not create object of interface.
- More than one interface can be implemented in a single class.
- A class implements an interface using implements keyword.
- If a class is implementing an interface it has to define all the methods given in that interface.
- If a class does not implement all the methods declared in the interface, the class must be declared abstract.
- The method signature for the method in the class must match the method signature as it appears in the interface.
- Any class can use an interface’s constants from the name of the interface like Test::roll.
- Classes that implement an interface can treat the constants as they were inherited.
- An interface can extend (inherit) an interface.
- One interface can inherit another interface using extends keywords.
- An Interface can not extends classes.
Defining Interface:
Syntax: -
interface interface_name
{
const properties;
Method;
}
Ex: -
interface Father
{
const a;
public function disp( );
}
Extending Interface:
- An interface can extend (inherit) an interface.
- One interface can inherit another interface using extends keywords.
- An Interface can not extends classes.
One Interface extending one Interface:
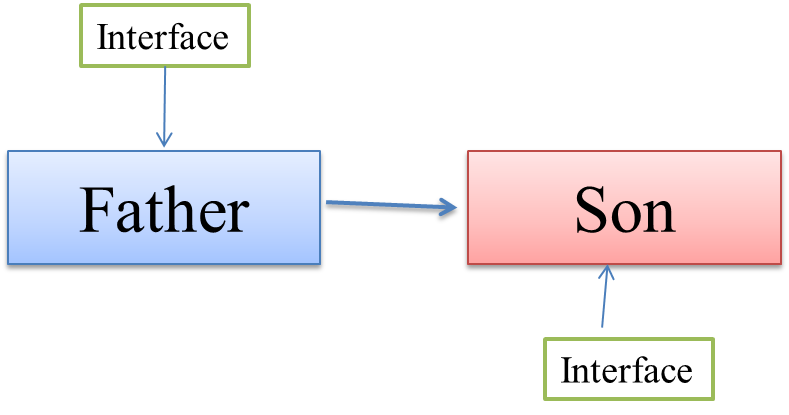
interface interface_name1
{
const properties;
Methods;
}
interface interface_name extends interface_name1
{
const properties;
Method;
}
One interface can extend more than one interface:
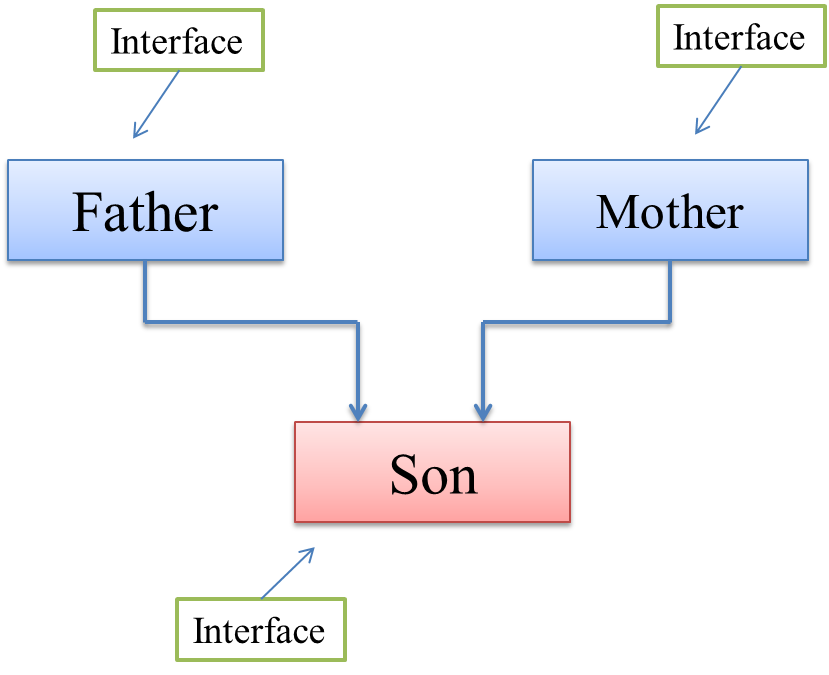
interface Father
{
}
interface Mother
{
}
interface Son extends Father, Mother
{
}
Implementing Class:
- More than one interface can be implemented in a single class.
- A class implements an interface using implements keyword.
- If a class is implementing an interface it has to define all the methods given in that interface.
- If a class does not implement all the methods declared in the interface, the class must be declared abstract.
- The method signature for the method in the class must match the method signature as it appears in the interface.
- Any class can use an interface’s constants from the name of the interface like Test::roll.
- Classes that implement an interface can treat the constants as they were inherited.
one interface can be implemented in a single class:
interface interface_name
{
const properties;
Methods;
}
class class_name implements interface_name
{
Properties;
Methods;
}
More than one interface can be implemented in a single class:
{
}
interface Mother
{
}
class Son implements Father, Mother
{
}
Abstract Class:
A class that is declared with abstract keyword, is known as abstract class in PHP. It can have abstract and non-abstract methods. It needs to be extended and its method implemented. objects of an abstract class cannot be created.
abstract class Test
{
}
Abstract Method:
A method that is declared as abstract and does not have implementation is known as abstract method
abstract function disp( ); //no body and abstract
abstract class Father
{
abstract function disp ( );
}
Class Son extends Father
{
public function disp ( )
{
echo “Abstract defined”;
}
}
Rules:
- We cannot use abstract classes to instantiate objects directly.
- objects of an abstract class cannot be created.
- The abstract methods of an abstract class must be defined in its subclass.
- If there is any abstract method in a class, that class must be abstract.
- A class can be abstract without having abstract method.
- It is not necessary to declare all methods abstract in a abstract class.
- We cannot declare abstract constructors or abstract static methods.
- If you are extending any abstract class that have abstract method, you must either provide the implementation of the method or make this class abstract.
Difference between interface and abstract class:
- An abstract class can have only abstract methods or only non-abstract methods or both, but All methods of an interface are abstract by default.
- An abstract class can declare properties/methods with access specifier, but interface can declare only constant properties and methods are by default abstract and public.
- A class can inherit only one abstract class and multiple inheritance is not possible for abstract class but A class can implement more than one interface and can achieve multiple inheritance.
- If a class contains even a single abstract method that class must be declared as abstract.
- In an abstract class, you can defined as well as It’s body methods but in the interface you can only define your methods.